6.1. pyopus.design.sensitivity
— Finite difference sensitivity computation and parameter screening¶
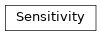
Sensitivity analysis (PyOPUS subsystem name: SENS)
Uses finite differences to estimate the sensitivities of performance measures to input parameters.
Parameter screening is possible based on sensitivity results.
-
class
pyopus.design.sensitivity.
Sensitivity
(evaluator, paramDesc, paramNames=None, debug=0, absPerturb=None, relPerturbHiLo=None, relPerturbValue=None, clipping=True, diffType='central', spawnerLevel=1)[source]¶ Callable object that computes sensitivities.
See
PerformanceEvaluator
for details on evaluator.The parameters are given by a dictionary paramDesc where parameter name is the key. One entry in this dictionary corresponds to one parameter. Every entry is a dictionary with the following members
lo .. lower bound (no lower bound if omitted).
hi .. upper bound
init .. parameter value where the sensitivity will be computed (optional)
paramDesc can be a list in which case the complete set of parameters is the union of parameters specified by list memebers.
paramNames specifies the order of parameters in the sensitivity vector. If not specified a default parameter ordering is constructed and stored in the
paramNames
member.Setting debug to a value greater than 0 turns on debug messages. The verbosity is proportional to the specified number.
diffType is the finite difference type (
central
,forward
, orbackward
)absPerturb specifies the absolute perturbation. If it is a single value it applies to all parameters. If it is a dictionary it applies to parameters listed in the dictionary.
relPerturbHiLo specifies the relative perturbation with respect to the allowed parameter span given by
lo
andhi
members of the paramDesc dictionary. If it is a single value it applies to all parameters for which a finitelo
andhi
value are specified. If it is a dictionary it applies only to parameters listed in the dictionary (provided they have finitelo
andhi
specified).relPerturbValue specified teh relative perturbation with respect to the parameter value. If it is a single value it applies to all parameters with nonzero value. If it is a dictionary it applies to parameters listed in the dictionary (provided they have nonzero value).
If clipping is set to
True
the perturbed values are verified againstlo
andhi
. If a bound is violated the finite difference type for that parameter is change so that the violation is remedied.If spawnerLevel is not greater than 1 the evaluations of perturbed points are dispatched to available computing nodes.
The number of circuit evaluations is stored in the
neval
member.Calling convention: obj(x0) where x0 is a dictionary of parameter values specifying the point where the sensitivity should be computed. If omitted the values in the
init
member of the paramDesc are used.Returns a tuple with performance deltas as the first member, the parameter perturbations as the second member, and the analysisCount dictionary as the third member.
The performance deltas are returned in a double dictionary where the first index is the performance measure name and the second index is the corner name. Every member is a list of values coresponding to parameters ordered as specified by paramNames.
If the computation of a performance delta for some parameter perturbation fails (i.e. any of the points required for computing the performance delta fail to produce a valid result) the corresponding entry in the list is
None
.Dividing performance deltas by parameter perturbations results in the sesitivities. This holds regardless of the type of perturbation specified with diffType.
The performance deltas are stored in the results member. The parameter perturbations are stored in the delta member.
Objects of this type store the number of analyses performed during the last call to the object in the analysisCount member.
-
diffVector
(measureName, cornerName)[source]¶ Returns the vector of performance differences in the order specified by parameterNames for the given measureName and cornerName. If the performance difference is an array (vector measures) the first index in the returned array is the parameter index while the remaining indices correspond to performance measure’s indices.
If some performance difference is not computed due to errors the corresponding components of the returned array are set to
np.nan
.If all performance measures fail to be computed for all parameters the size of the array is unknown. In that case an empty array is returned.
-
pyopus.design.sensitivity.
screen
(diffVector, delta, paramNames=None, contribThreshold=0.01, cumulativeThreshold=0.25, useSens=False, squared=True, debug=0)[source]¶ Performs parameter screening. Returns two lists of inidices corresponding to parameters whose influence is below threshold and parameters whose influsence is above threshold. The indices are ordered by absolute sensitivity from the smallest to the largest.
diffVector is the vector of performance differences corresponding to perturbations of parameters specified by paramNames. Only scalar performances can be screened (i.e. this must be a 1-D vector).
If paramNames is not specified parameters indices starting with 0 are used in the debug output.
delta is the vector of parameter perturbations.
A parameter is considered to have low influence if its relative contribution is below contribThreshold and the relative cumulative contribution of the set of all parameters with low influence does not exceed cumulativeThreshold.
If useSens is set to
True
the performance measure sensitivities are used instead of deltas. This makes sense only if all parameters are expressed in the same units.If squared is set to
False
the absolute sensitivities/deltas are used instead of squared sensitivities/deltas in the computation of the relative influence.